Debugging is an essential skill for every developer, and when it comes to JavaScript, Chrome DevTools is one of the most powerful tools at your disposal. Whether you’re dealing with unexpected errors or trying to optimize your code, DevTools provides a suite of features that can make your life easier.
Table of Contents
ToggleWhy Use Chrome DevTools?
Pros:
- Free and Built-In: Chrome DevTools is available in the Chrome browser, eliminating the need for additional software.
- Feature-Rich: It offers a variety of tools for debugging, performance analysis, and even design tweaks.
- Live Editing: You can make changes directly in the browser and see the results immediately.
Cons:
- Chrome Dependency: You’ll need Chrome, which may not reflect issues specific to other browsers.
- Steep Learning Curve: While powerful, the vast array of features can be overwhelming for beginners.
Getting Started with DevTools
Opening Chrome DevTools
You can open DevTools in several ways:
- Right-click on a page and select Inspect.
- Use the shortcut
Ctrl+Shift+I
(Windows/Linux) orCmd+Option+I
(Mac). - Go to the Chrome menu > More Tools > Developer Tools.
Once open, navigate to the Console and Sources tabs to start debugging.
Understanding the Basics
The Console Tab
The Console is your go-to place for:
- Viewing errors and warnings.
- Executing JavaScript snippets.
- Logging messages using
console.log()
.
Pro Tip: Use console.table()
for visualizing arrays and objects in a tabular format.
Potential Pitfall: Over-reliance on console.log()
can clutter your code and make debugging less efficient.
Setting Breakpoints
Breakpoints allow you to pause your code at specific lines and inspect its state. Here’s how to set them:
- Open the Sources tab.
- Click on the line number where you want to pause execution.
- Reload the page or trigger the event that executes the code.
Advantages:
- Inspect variables and their values in real-time.
- Step through code line by line.
Drawback: Breakpoints can be tricky to manage in large files, especially without proper organization.
Debugging Tips
1. Step Through Your Code
Use the controls in the Sources tab to:
- Step Over: Skip functions without stepping into them.
- Step Into: Dive into functions line by line.
- Step Out: Exit the current function and return to the caller.
Caution: Avoid stepping too quickly; you might miss important changes in state.
2. Watch Variables
The Watch panel allows you to monitor specific variables. To add a variable:
- Right-click in the Watch section.
- Enter the variable name.
3. Use Conditional Breakpoints
To avoid unnecessary pauses, set breakpoints with conditions:
- Right-click on a line number.
- Select Add conditional breakpoint.
- Enter a condition (e.g.,
x > 10
).
4. Explore the Scope and Call Stack
The Scope section displays variables available in the current context.
The Call Stack shows the sequence of functions leading to the current execution point.
Advanced Features
Network Tab
Use the Network tab to analyze API requests and responses. This is invaluable for debugging issues with fetch calls or AJAX.
Performance Tab
Identify performance bottlenecks in your code using this tab.
Limitations of Chrome DevTools
While powerful, DevTools has some limitations:
- Browser-Specific: Issues occurring in non-Chrome browsers may require alternative tools.
- Real-Time Debugging Only: DevTools doesn’t provide static analysis of your code.
Final Thoughts
Chrome DevTools is a must-have for any JavaScript developer. By mastering its features, you can efficiently debug your code and improve your workflow. Start with the basics, and as you grow comfortable, explore the advanced tools to unlock the full potential of DevTools.
You may also like:
1) How do you optimize a website’s performance?
2)Â Change Your Programming Habits Before 2025: My Journey with 10 CHALLENGES
3)Â Senior-Level JavaScript Promise Interview Question
4)Â What is Database Indexing, and Why is It Important?
5)Â Can AI Transform the Trading Landscape?
Read more blogs from Here
What are your favorite features in Chrome DevTools? Let me know in the comments below!
Follow me on Linkedin
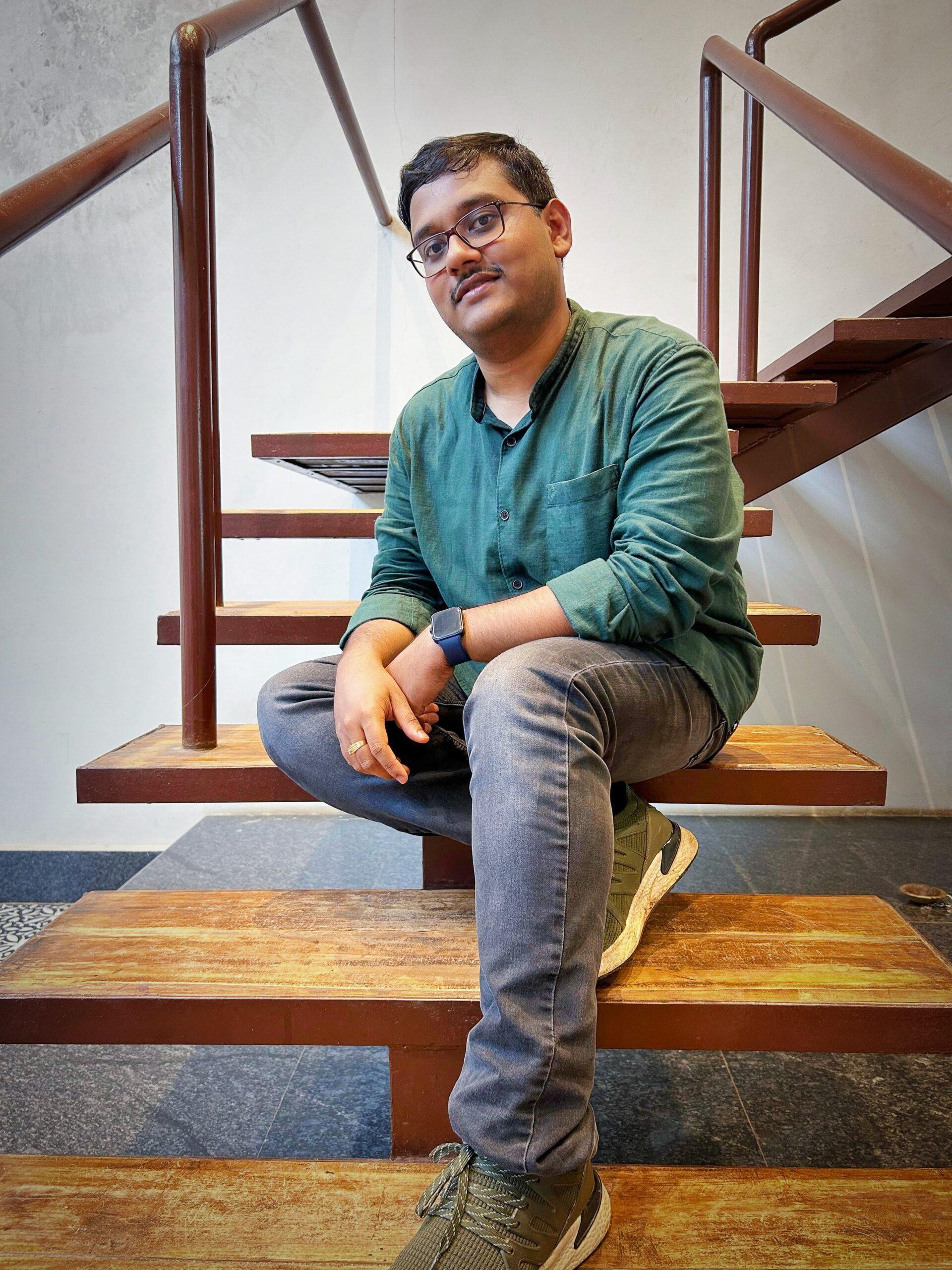
Trust me, I’m a software developer—debugging by day, chilling by night.