As Node.js continues to be a leading framework for developing high-performance web applications, security concerns have grown alongside its popularity. Web applications are a prime target for attackers, and ignoring security flaws can lead to catastrophic outcomes like data breaches, service downtime, or reputational damage.
Table of Contents
Toggle1. Injection Attacks
What is it?
Injection attacks, like SQL injection or command injection, occur when an attacker sends untrusted input to an interpreter, tricking it into executing malicious commands.
How to Protect:
- Use Parameterized Queries: Avoid constructing SQL queries with string interpolation. Use libraries like
pg
for PostgreSQL ormongoose
for MongoDB to sanitize queries. - Validate Input: Use libraries like
Joi
orexpress-validator
to validate and sanitize user input. - Avoid eval(): Never use
eval()
or similar functions (Function()
,setTimeout
with strings) in your code.
2. Cross-Site Scripting (XSS)
What is it?
XSS allows attackers to inject malicious scripts into web pages viewed by other users, potentially stealing session cookies, hijacking accounts, or defacing websites.
How to Protect:
- Escape User Input: Use libraries like
DOMPurify
on the client side andsanitize-html
on the server side to sanitize data before rendering. - Use a CSP: Implement a Content Security Policy (CSP) to block unauthorized scripts from executing.
- Template Engines: Use secure template engines like
Pug
orEJS
that automatically escape HTML.
3. Cross-Site Request Forgery (CSRF)
What is it?
CSRF exploits users’ authenticated sessions by making unauthorized requests on their behalf.
How to Protect:
- Use CSRF Tokens: Middleware like
csurf
generates tokens that must be included in all state-changing requests. - Check Referer Header: Validate the origin of incoming requests.
- Use SameSite Cookies: Mark cookies as
SameSite=strict
orSameSite=lax
to prevent them from being sent with cross-origin requests.
4. Insecure Dependencies
What is it?
Using outdated or vulnerable npm packages exposes applications to known exploits.
How to Protect:
- Regular Updates: Periodically update dependencies using
npm audit
or tools likedependabot
. - Audit Dependencies: Run
npm audit fix
to identify and resolve vulnerabilities. - Limit Dependencies: Avoid unnecessary dependencies by evaluating libraries’ trustworthiness and code quality.
5. Improper Error Handling
What is it?
Detailed error messages can leak sensitive information such as database schemas, API keys, or server architecture.
How to Protect:
- Use Generic Error Messages: Hide sensitive data in production error messages.
- Centralized Error Handling: Create a centralized error-handling middleware to manage exceptions without leaking critical details.
- Log Securely: Store detailed error logs in secure storage for internal access only.
6. Lack of Rate Limiting
What is it?
An absence of rate-limiting mechanisms exposes applications to brute force attacks or denial of service (DoS).
How to Protect:
- Implement Rate Limiting: Use libraries like
express-rate-limit
to restrict request frequency. - CAPTCHA: Implement CAPTCHAs for critical endpoints like login or registration.
- Use Load Balancers: A load balancer with built-in DoS protection, like AWS Elastic Load Balancer, adds an extra layer of security.
7. Server-Side Request Forgery (SSRF)
What is it?
SSRF exploits vulnerabilities that allow attackers to make unauthorized requests to internal systems.
How to Protect:
- Whitelist Allowed Domains: Restrict external requests to a predefined list of trusted domains.
- Avoid Direct User Input: Never use user input directly in requests without validation.
- Monitor Traffic: Use a WAF (Web Application Firewall) to detect and block SSRF attempts.
8. Insufficient Authentication and Authorization
What is it?
Weak authentication mechanisms or missing authorization checks allow attackers to gain unauthorized access to resources.
How to Protect:
- Use Strong Password Policies: Enforce strong passwords using libraries like
zxcvbn
. - Implement MFA: Require multi-factor authentication for sensitive actions.
- Least Privilege Principle: Minimize access permissions for users and services to only what is required.
9. Using Insecure Configurations
What is it?
Improper server or app configurations, such as running in debug mode or exposing environment variables, can introduce vulnerabilities.
How to Protect:
- Secure Environment Variables: Store secrets in a secure vault like AWS Secrets Manager or HashiCorp Vault.
- Disable Unnecessary Features: Turn off features not in use, such as directory listing or default error pages.
- Use HTTPS: Enforce HTTPS for all connections to prevent data interception.
Final Thoughts
Securing your Node.js web application requires a proactive and layered approach. Stay informed, regularly audit your codebase, and keep your dependencies up to date.
You may also like:
1) How do you optimize a website’s performance?
2)Â Change Your Programming Habits Before 2025: My Journey with 10 CHALLENGES
3)Â Senior-Level JavaScript Promise Interview Question
4)Â What is Database Indexing, and Why is It Important?
5)Â Can AI Transform the Trading Landscape?
Read more blogs from Here
Share your experiences in the comments, and let’s discuss how to tackle them!
Follow me on Linkedin
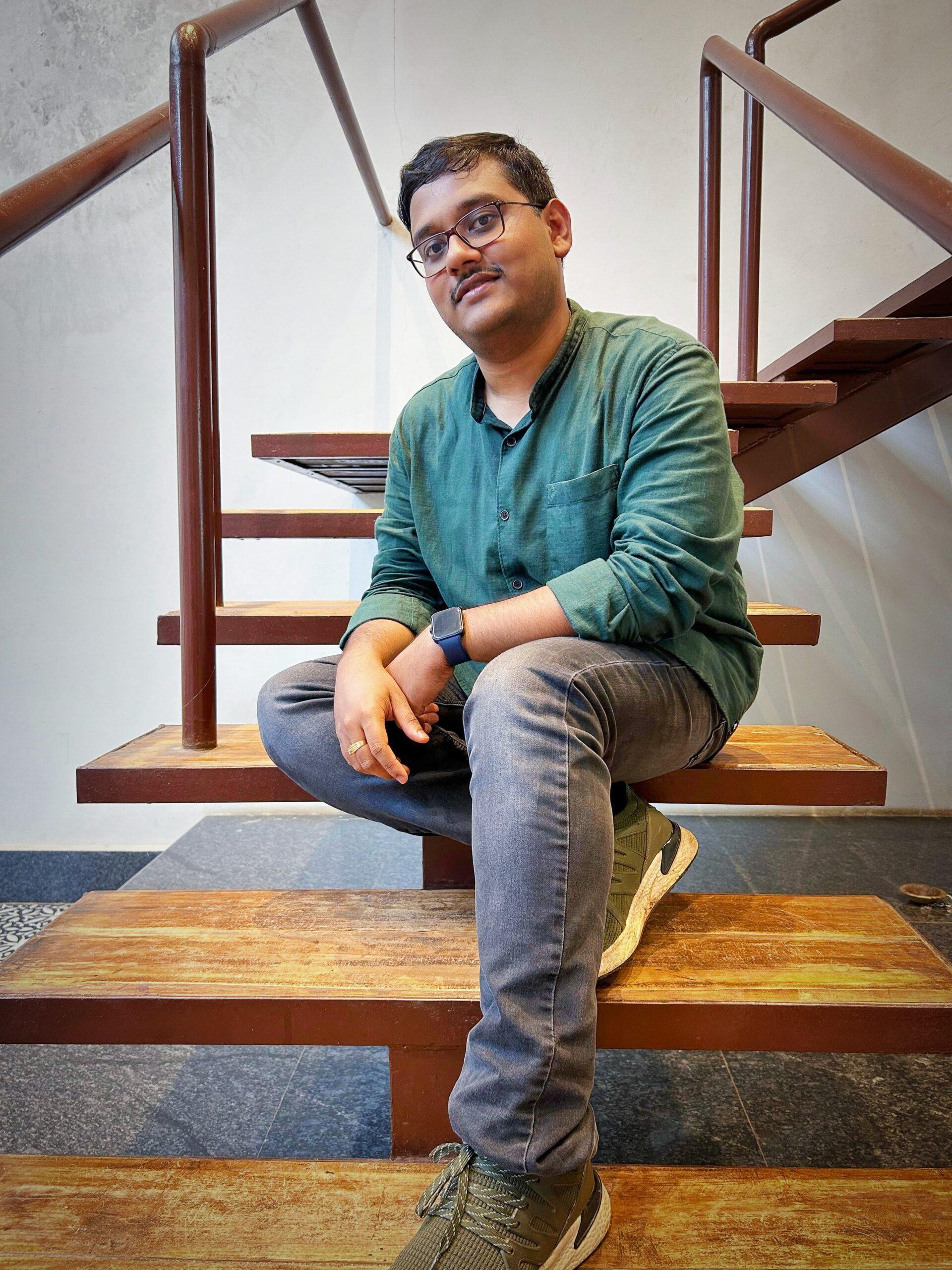
Trust me, I’m a software developer—debugging by day, chilling by night.