In the world of front-end development, writing clean, scalable, and maintainable CSS can sometimes be challenging, especially as projects grow. This is where CSS preprocessors come in. They bring extra power and flexibility to CSS, making it easier for developers to manage complex stylesheets.
Table of Contents
ToggleWhat Is a CSS Preprocessor?
A CSS preprocessor is a scripting language that extends the default capabilities of CSS. With preprocessors, you can write CSS in a more functional, modular, and organized way, and then compile this preprocessed code into regular CSS files that browsers can read.
Popular CSS preprocessors include:
- Sass (Syntactically Awesome Style Sheets): One of the oldest and most widely used preprocessors.
- LESS: Known for its simplicity and flexibility.
- Stylus: Offers a highly flexible syntax.
Why Use CSS Preprocessors?
Here are some key reasons to consider adding a CSS preprocessor to your toolkit:
1. Variables for Reusable Values
In standard CSS, you must manually repeat values (like colors or font sizes) throughout your stylesheet. Preprocessors allow you to define variables, which act like placeholders for these values. This makes it easier to change a value in one place and have it reflected across the entire codebase.
Example:
$primary-color: #3498db;
$font-stack: Helvetica, sans-serif;
body {
font-family: $font-stack;
color: $primary-color;
}
2. Nesting for Hierarchical Structure
CSS preprocessors allow you to nest CSS rules within each other. Nesting makes the relationship between styles more intuitive and mirrors the HTML structure. This hierarchical structure improves readability and organization.
Example:
nav {
ul {
margin: 0;
padding: 0;
list-style: none;
}
li {
display: inline-block;
margin-right: 10px;
}
a {
text-decoration: none;
color: #333;
}
}
3. Partials for Modular Code
With preprocessors, you can break CSS into partials (smaller files) that can be imported into a main stylesheet. This modular approach keeps styles organized and allows for better reusability across multiple pages or components.
Example:
// _buttons.scss
.button {
padding: 10px 20px;
border: none;
border-radius: 5px;
}
// main.scss
@import 'buttons';
4. Mixins for Reusable Code Blocks
A mixin is a block of code that can be reused throughout the stylesheet. It can accept arguments, making it a powerful tool for creating flexible and reusable styles. This is especially useful for repetitive code, such as vendor prefixes or complex animations.
Example:
@mixin border-radius($radius) {
-webkit-border-radius: $radius;
-moz-border-radius: $radius;
-ms-border-radius: $radius;
border-radius: $radius;
}
.button {
@include border-radius(10px);
}
5. Inheritance for Avoiding Code Duplication
Preprocessors support inheritance, allowing you to extend existing styles. This is beneficial for avoiding redundancy and keeping your code DRY (Don’t Repeat Yourself).
Example:
%default-box {
padding: 20px;
border: 1px solid #ddd;
background-color: #f9f9f9;
}
.box {
@extend %default-box;
border-radius: 10px;
}
6. Mathematical Operations for Dynamic Styling
CSS preprocessors allow you to perform math operations on properties like widths, heights, and margins. This is useful for calculating values dynamically, especially for fluid and responsive designs.
Example:
.container {
width: 100% / 3;
margin: 10px * 2;
}
Advantages of Using CSS Preprocessors
- Enhanced Productivity: Preprocessors enable developers to write CSS faster and more efficiently.
- Improved Organization: Nesting, partials, and mixins help keep your code modular and organized.
- Easier Maintenance: Variables and mixins reduce redundancy, making it easier to update styles across a large project.
- Cross-Browser Compatibility: Mixins allow you to include vendor prefixes and other browser-specific rules with minimal effort.
- Faster Development: With features like variables and inheritance, you spend less time writing repetitive code.
Disadvantages of CSS Preprocessors
Despite their benefits, CSS preprocessors do come with some potential drawbacks:
- Learning Curve: If you’re new to CSS preprocessors, learning the syntax and workflow can take time.
- Dependency on Compilation: Preprocessor code needs to be compiled into standard CSS, adding an extra build step.
- Performance Overhead: If not used wisely, excessive nesting or complex mixins can create bloated CSS, impacting performance.
Conclusion
CSS preprocessors, like Sass and LESS, provide essential features for modern CSS development, from variables to modular code structures. While they require a bit of setup and learning, the benefits they offer in terms of efficiency, maintainability, and readability are worth it, especially for larger projects. By adopting CSS preprocessors, you can streamline your workflow and write cleaner, more manageable CSS.
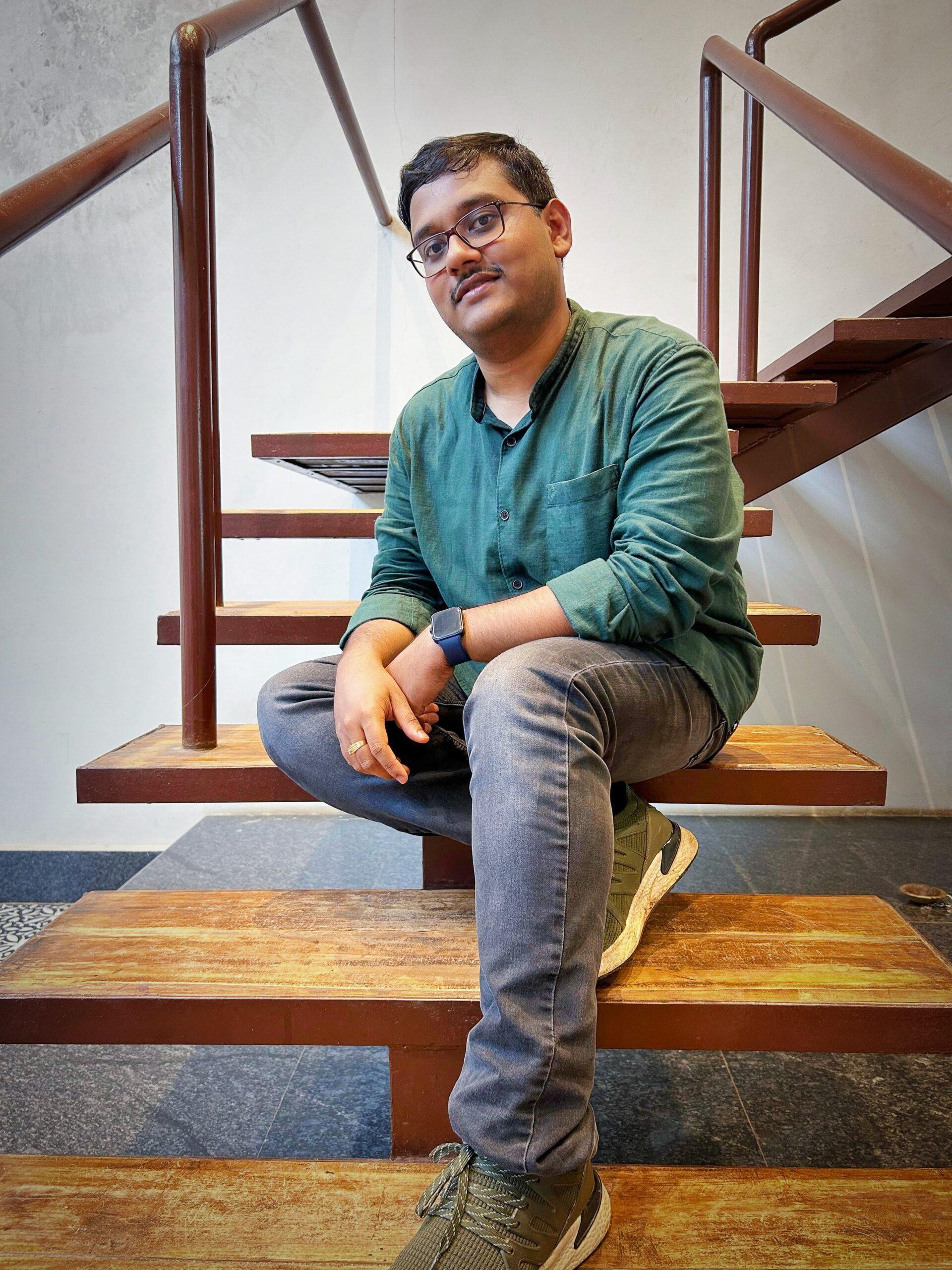
Trust me, I’m a software developer—debugging by day, chilling by night.