The Document Object Model (DOM) is a crucial concept in web development, serving as a bridge between HTML and the interactive features that JavaScript can add to websites. Without the DOM, websites would remain static, lacking the dynamic interactivity that users have come to expect. This article explores what the DOM is, how it works, and why it’s foundational for modern web development.
1. Understanding the DOM: Definition and Purpose
At its core, the DOM is a programming interface provided by browsers to represent a webpage’s content, structure, and style. It’s essentially an organized, hierarchical representation of a document, typically structured in HTML or XML. The DOM allows programming languages, notably JavaScript, to manipulate the content, structure, and styling of a webpage dynamically.
When a web page loads, the browser parses the HTML and CSS and creates a live model of the page that can be modified using JavaScript. This model is the DOM. This approach lets developers change elements, attributes, and CSS properties on the fly without reloading the page, leading to smoother, more interactive user experiences.
The DOM is also cross-platform and language-independent. It’s not tied to JavaScript, even though JavaScript is the most commonly used language to manipulate it. Technically, any language that supports the DOM API (Application Programming Interface) can interact with the document.
2. The Structure of the DOM
The DOM is often visualized as a tree structure, where each HTML or XML element is represented as a “node” in this tree. Here’s how it generally breaks down:
- Document Node: This is the root of the DOM tree and represents the entire document. It’s the entry point through which scripts can access and manipulate the document content.
- Element Nodes: Each HTML element, such as
<div>
,<p>
,<h1>
, etc., is an element node in the tree. These nodes are nested within one another based on the HTML structure. - Attribute Nodes: Attributes like
class
,id
, andhref
associated with HTML elements are attribute nodes. They provide additional properties for element nodes. - Text Nodes: These nodes represent the actual text within HTML elements. For example, in
<p>Hello World</p>
, “Hello World” is a text node inside the<p>
element node.
The structure of the DOM tree is hierarchical, with each element potentially containing other elements or text, creating a parent-child relationship. Here’s an example of a simple HTML document and how it would be structured in the DOM:
Sample Page
Welcome to the DOM
This is a simple example.
This document would be represented in the DOM as a hierarchy, with html
as the root node, head
and body
as its children, and so on. Each node can have children (nested elements) or siblings (elements on the same level).
3. How the DOM Works: Parsing and Rendering
The process begins with the parsing of the HTML and CSS files by the browser to create the DOM and CSSOM (CSS Object Model) trees, respectively. These two trees are then combined to form the Render Tree, which defines the visual display of elements on the screen.
- Step 1: HTML Parsing – The browser parses the HTML document and builds a tree-like structure (DOM Tree) that represents the document’s structure.
- Step 2: CSS Parsing – Simultaneously, CSS files are parsed to build the CSSOM. This tree specifies the styles associated with elements.
- Step 3: Render Tree Construction – The DOM and CSSOM are combined to form the Render Tree, which dictates how elements will be visually presented.
- Step 4: Layout and Paint – The browser calculates the exact positions and dimensions of each element (layout) and then paints the elements on the screen.
Any JavaScript that modifies the DOM or CSSOM after this process triggers a reflow (layout recalculation) or repaint (visual update). Minimizing these operations is essential for maintaining performance, particularly on complex, dynamic web pages.
4. Manipulating the DOM with JavaScript
One of the primary reasons the DOM is so powerful is because it allows for dynamic interaction with JavaScript. JavaScript can add, remove, modify, or even completely rearrange elements in the DOM. Here are some common methods and properties used to interact with the DOM:
Accessing Elements:
document.getElementById()
: Selects an element by its ID.document.getElementsByClassName()
: Selects elements by their class.document.getElementsByTagName()
: Selects elements by their tag name.document.querySelector()
anddocument.querySelectorAll()
: Select elements using CSS selectors.
Creating and Adding Elements:
document.createElement()
: Creates a new element.parentNode.appendChild(newNode)
: Appends a new child element to a parent node.parentNode.insertBefore(newNode, referenceNode)
: Inserts an element before a specified reference node.
Removing Elements:
parentNode.removeChild(childNode)
: Removes a specified child from a parent node.
Modifying Attributes and Styles:
element.setAttribute()
: Sets an attribute on an element.element.style
: Modifies inline styles directly.
Event Handling:
- The DOM also supports event handling, allowing developers to add interactivity. For instance,
element.addEventListener('click', callback)
allows you to specify a function to be run when an element is clicked.
- The DOM also supports event handling, allowing developers to add interactivity. For instance,
Example:
// Changing text in a element with id="demo"
document.getElementById("demo").innerText = "Hello, DOM!";
This example dynamically changes the text inside a <p>
element. Similarly, the DOM API offers methods to make elements disappear, change colors, or trigger animations in response to user actions.
5. The DOM and Performance
Interacting with the DOM can be computationally expensive, especially if the document is large or complex. Here are a few performance tips:
- Minimize Reflows and Repaints: Adding or removing nodes frequently can cause reflows and repaints, which may slow down page performance. Batch DOM updates or use CSS classes to apply multiple style changes at once.
- Use Document Fragments: When adding multiple elements to the DOM, use
DocumentFragment
to add them all at once, which is more efficient. - Optimize Event Handling: Avoid adding event listeners to many individual elements; instead, use event delegation to add a single listener to a parent element.
6. The DOM in Modern JavaScript Frameworks
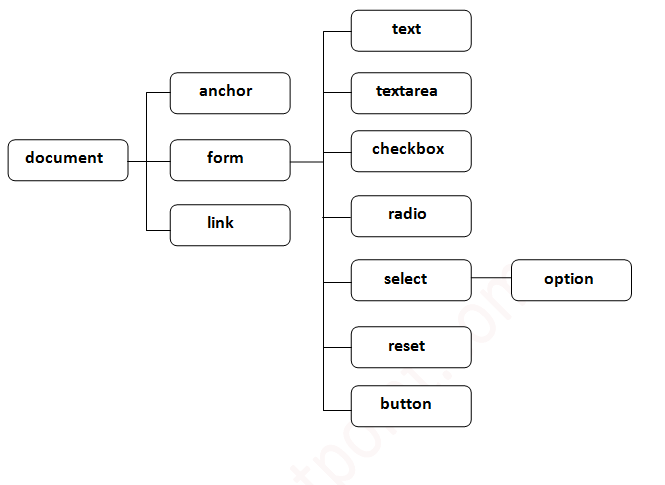
JavaScript frameworks like React, Vue, and Angular interact with the DOM differently by using something called the Virtual DOM. The Virtual DOM is a lightweight copy of the DOM that exists purely in memory. Instead of updating the DOM directly, these frameworks update the Virtual DOM. They then compare it to the actual DOM and only apply changes where necessary. This approach greatly improves performance by reducing the number of direct DOM manipulations.
For example, React’s Virtual DOM allows it to perform “diffing,” a process where it determines the minimal number of updates needed and only changes those parts of the actual DOM. This makes applications faster and more efficient.
7. Conclusion
The Document Object Model (DOM) is a foundational concept in web development. It provides a structured, programmable interface for web content, making it possible for developers to create interactive, dynamic websites. Through the DOM, JavaScript can manipulate web pages in real time, responding to user interactions, changing content, and altering the page’s style.
Understanding how the DOM works, how to manipulate it efficiently, and its implications for performance are essential skills for web developers. As JavaScript frameworks evolve and improve, knowing the basics of the DOM remains crucial, even in the context of advanced technologies like the Virtual DOM. By mastering DOM manipulation, developers unlock the potential to create more interactive, responsive, and engaging user experiences.