APIs are the backbone of modern applications, enabling communication between diverse systems and devices. However, when dealing with high-traffic APIs, one of the biggest challenges is ensuring availability while preventing abuse. Rate limiting and abuse prevention strategies play a crucial role in managing these concerns effectively.
What Is API Rate Limiting?
Rate limiting is the process of controlling how often a client can make requests to an API within a specified period. This mechanism helps protect APIs from being overwhelmed by excessive traffic, whether intentional (e.g., DDoS attacks) or unintentional (e.g., buggy client code).
Why Is Rate Limiting Important?
- Prevents Resource Exhaustion: Protects servers from being overwhelmed, ensuring consistent performance for all users.
- Enhances Security: Limits malicious actors from spamming endpoints.
- Improves Fairness: Ensures all users have equitable access to the API.
- Optimizes Costs: Controls overuse of resources to reduce operational costs.
Strategies for Rate Limiting and Abuse Prevention in Node.js
1. Using Middleware
Node.js offers several middleware options, such as express-rate-limit, to implement rate limiting. Here’s a simple implementation:
const express = require('express');
const rateLimit = require('express-rate-limit');
const app = express();
// Define rate limiting rules
const limiter = rateLimit({
windowMs: 15 * 60 * 1000, // 15 minutes
max: 100, // Limit each IP to 100 requests per windowMs
message: 'Too many requests, please try again later.',
});
// Apply the rate limiting middleware to all API routes
app.use('/api', limiter);
app.get('/api/resource', (req, res) => {
res.send('This is a rate-limited resource.');
});
app.listen(3000, () => console.log('Server running on port 3000'));
2. IP-Based Throttling
You can use packages like express-rate-limit
or redis
to track request counts based on client IPs. Redis is especially useful for distributed environments.
3. Token Bucket Algorithm
The token bucket algorithm ensures smooth request handling by allowing clients to “borrow” tokens for future requests. Libraries like bottleneck
can help implement this in Node.js.
4. Request Queueing
Queuing excessive requests prevents sudden spikes. Use packages like bull
for advanced request queuing. Example:
const Queue = require('bull');
const apiQueue = new Queue('api-queue');
apiQueue.process(async (job) => {
// Handle API request here
return 'Request processed successfully.';
});
// Add requests to the queue
app.post('/api/resource', async (req, res) => {
const job = await apiQueue.add({});
res.status(202).send(`Your request is queued. Job ID: ${job.id}`);
});
5. API Key Validation
Enforce client-side API keys and apply rate limits per key to avoid overuse by specific users. Middleware libraries like express-api-key
can simplify API key validation.
6. Honeypot Techniques
Expose certain endpoints as honeypots that trap bots or abusive clients and add their IPs to a deny list.
7. Advanced Behavioral Analytics
Implement machine learning or rule-based systems to detect patterns indicative of abuse. Analyze metrics like IP switching, unusual request frequency, or suspicious headers.
8. Dynamic Rate Limiting
Adjust rate limits dynamically based on factors like user subscription plans, current server load, or time of day. This can be implemented using Redis and logic tailored to your business needs.
Scaling Strategies for High-Traffic APIs
When APIs experience high traffic, scaling becomes imperative. Here are a few strategies:
Horizontal Scaling
Deploy multiple instances of your Node.js application and use load balancers like Nginx to distribute traffic.
Global Caching
Utilize caching layers like Redis, Memcached, or services like Cloudflare to serve repeat requests without hitting the backend.
Distributed Rate Limiting
Synchronize request counters across multiple server instances using Redis or a similar tool.
Monitoring and Metrics
To ensure your rate-limiting mechanisms are effective, implement robust monitoring tools. Use:
- Log Analysis: Use tools like ELK Stack or Datadog to monitor logs.
- APM Tools: Application Performance Monitoring (APM) tools like New Relic or AppDynamics to track response times and errors.
- Custom Dashboards: Build dashboards with metrics like requests per minute, blocked requests, and server resource utilization.
Conclusion
Implementing API rate limiting and abuse prevention strategies in Node.js is critical for managing high-traffic APIs. With middleware tools, algorithmic strategies, and robust monitoring, you can ensure your API remains reliable and secure.
You may also like:
1) How do you optimize a website’s performance?
2) Change Your Programming Habits Before 2025: My Journey with 10 CHALLENGES
3) Senior-Level JavaScript Promise Interview Question
4) What is Database Indexing, and Why is It Important?
5) Can AI Transform the Trading Landscape?
Read more blogs from Here
Share your experiences in the comments, and let’s discuss how to tackle them!
Follow me on Linkedin
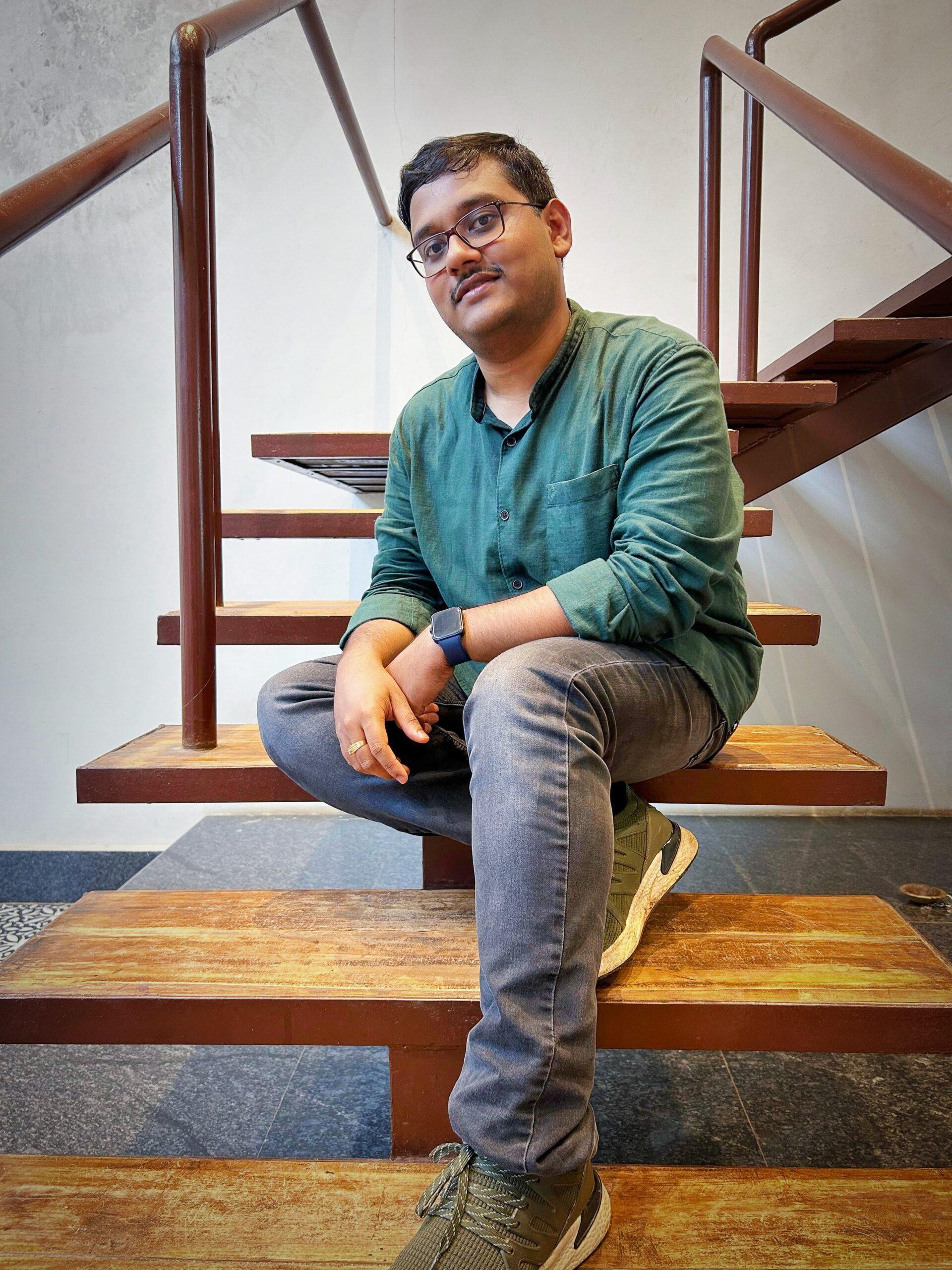
Trust me, I’m a software developer—debugging by day, chilling by night.