In today’s digital era, real-time applications are everywhere—from live chat systems and collaborative tools to dashboards and IoT systems. Implementing real-time data synchronization between a client and server is a crucial feature for creating dynamic and interactive user experiences.
Why Real-Time Data Sync?
Real-time synchronization ensures that changes made in one place are immediately reflected everywhere. Common use cases include:
- Live Chats: Messages instantly show up for participants.
- Dashboards: Updated metrics are displayed in real time.
- Collaborative Tools: All users see updates simultaneously.
MongoDB, with its powerful change streams feature, combined with the event-driven capabilities of Node.js, makes implementing this functionality both seamless and efficient.
Setup: What We’ll Build
We’ll create a basic Node.js app where:
- Clients can update a MongoDB database.
- Other connected clients receive live updates whenever changes occur in the database.
Prerequisites
Before starting, ensure you have the following:
- Node.js installed
- MongoDB Atlas account (or a local MongoDB instance)
- Basic understanding of JavaScript, Node.js, and MongoDB
Step 1: Project Setup
Start by creating a new Node.js project:
mkdir realtime-sync
cd realtime-sync
npm init -y
npm install express mongoose socket.io dotenv
Folder Structure
Set up your project like this:
realtime-sync/
├── server.js
├── .env
└── models/
└── Item.js
Step 2: Connecting to MongoDB
Create a .env
file with your MongoDB URI:
MONGO_URI=mongodb+srv://:@cluster.mongodb.net/myDatabase?retryWrites=true&w=majority
In server.js
, connect to MongoDB:
const express = require("express");
const mongoose = require("mongoose");
const dotenv = require("dotenv");
dotenv.config();
mongoose
.connect(process.env.MONGO_URI, { useNewUrlParser: true, useUnifiedTopology: true })
.then(() => console.log("MongoDB connected"))
.catch((err) => console.error("MongoDB connection error:", err));
Step 3: Create a MongoDB Model
Create a basic model in models/Item.js
:
const mongoose = require("mongoose");
const ItemSchema = new mongoose.Schema({
name: { type: String, required: true },
value: { type: Number, required: true },
}, { timestamps: true });
module.exports = mongoose.model("Item", ItemSchema);
Step 4: Set Up the Server
Add the server logic in server.js
:
const app = express();
const http = require("http").createServer(app);
const io = require("socket.io")(http);
// Middleware
app.use(express.json());
app.use(express.urlencoded({ extended: true }));
// Serve a test route
app.get("/", (req, res) => {
res.send("Real-time Data Sync App");
});
// Socket.IO connection
io.on("connection", (socket) => {
console.log("Client connected:", socket.id);
socket.on("disconnect", () => {
console.log("Client disconnected:", socket.id);
});
});
const PORT = process.env.PORT || 3000;
http.listen(PORT, () => {
console.log(`Server running on port ${PORT}`);
});
Step 5: Integrate Real-Time Sync
MongoDB supports real-time data with change streams. Let’s use them!
Add this logic to server.js
:
const Item = require("./models/Item");
// Watch for changes in MongoDB
const changeStream = Item.watch();
changeStream.on("change", (change) => {
console.log("Change detected:", change);
// Notify all connected clients
io.emit("databaseChange", change);
});
// Add a route to create new items
app.post("/items", async (req, res) => {
try {
const newItem = await Item.create(req.body);
res.status(201).send(newItem);
} catch (err) {
console.error(err);
res.status(500).send("Error creating item");
}
});
Step 6: Client Implementation
For testing, create a simple HTML client.
index.html
Real-Time Data Sync
Real-Time Data Sync
How It Works
- Clients make changes through POST requests to
/items
. - MongoDB’s
changeStream
detects the change and notifies the server. - The server broadcasts updates to connected clients via Socket.IO.
- Clients update their UI in real time.
Testing the App
Start the server:
node server.js
Open
index.html
in a browser.Use tools like Postman or a similar API client to send a POST request:
POST /items
{
"name": "Sample Item",
"value": 42
}
You’ll see the real-time update reflected in the browser!
Optimizing for Scalability
- Replica Sets: MongoDB change streams require replica sets, even for development. Use MongoDB Atlas or a locally configured replica set.
- Load Balancing: Use tools like NGINX or HAProxy to distribute traffic across multiple instances.
- Namespace Channels: For large-scale apps, split real-time channels by functionality to reduce event noise.
Conclusion
With MongoDB change streams and Socket.IO, building real-time features is straightforward and efficient. This architecture can scale easily and supports a variety of use cases.
Real-time data synchronization isn’t just a trend—it’s becoming a necessity in modern applications. Start integrating it today to enhance the user experience of your app!
You may also like:
1) How do you optimize a website’s performance?
2) Change Your Programming Habits Before 2025: My Journey with 10 CHALLENGES
3) Senior-Level JavaScript Promise Interview Question
4) What is Database Indexing, and Why is It Important?
5) Can AI Transform the Trading Landscape?
Read more blogs from Here
Share your experiences in the comments, and let’s discuss how to tackle them!
Follow me on Linkedin
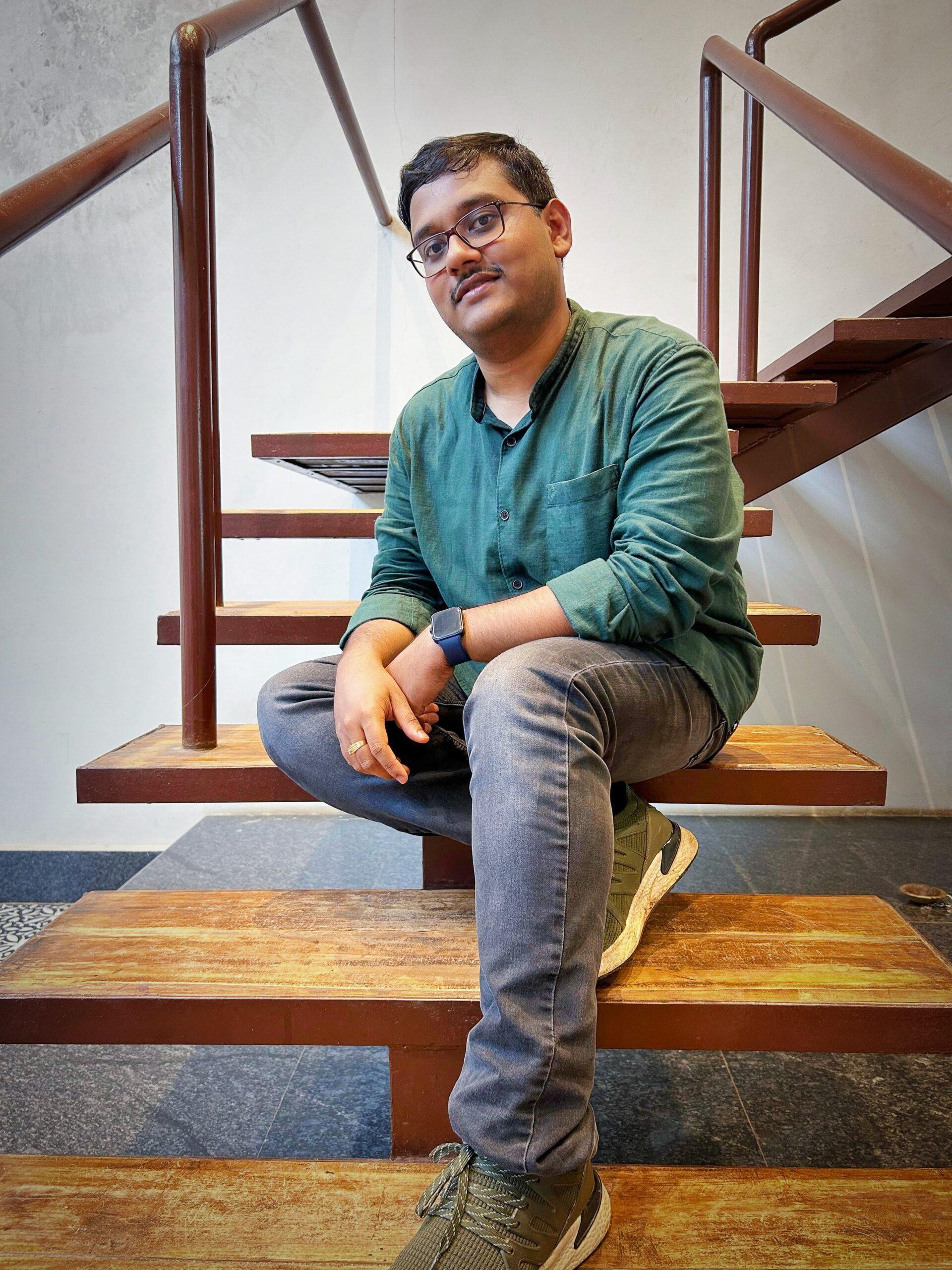
Trust me, I’m a software developer—debugging by day, chilling by night.