Node.js has become a popular choice for developing APIs due to its high performance, scalability, and extensive ecosystem. However, with great power comes the responsibility of ensuring your APIs are secure, especially when dealing with enterprise-level applications where data breaches can have severe consequences.
1. Implement Authentication and Authorization
Use Secure Authentication Mechanisms
Choose robust and well-supported libraries like Passport.js, OAuth2, or Auth0 to handle authentication. Avoid implementing your own authentication logic as it may introduce vulnerabilities.
- Use JSON Web Tokens (JWT) for stateless authentication, ensuring proper signing and expiration policies.
- Always hash passwords securely with libraries like bcrypt or argon2 before storing them.
Enforce Role-Based Access Control (RBAC)
Implement fine-grained access control to ensure users have access only to the resources they are authorized to use. RBAC can be enforced using libraries like Casbin or by creating middleware to manage roles and permissions.
2. Protect Against SQL/NoSQL Injection
Injection attacks are one of the most common vulnerabilities in APIs. To prevent them:
- Use parameterized queries or query builders like Sequelize or Knex.js for SQL databases.
- For NoSQL databases like MongoDB, validate and sanitize input fields using libraries such as mongoose-validator or validator.js.
3. Sanitize User Inputs
Validation and Sanitization Libraries
Incorporate libraries like Joi, Yup, or express-validator to validate input data and ensure that it meets expected formats and types.
const { body, validationResult } = require('express-validator');
app.post('/api/data', [
body('email').isEmail(),
body('age').isInt({ min: 1 })
], (req, res) => {
const errors = validationResult(req);
if (!errors.isEmpty()) {
return res.status(400).json({ errors: errors.array() });
}
res.send('Input is valid!');
});
4. Enable HTTPS
Always encrypt communications using HTTPS. This ensures data in transit is protected from eavesdropping and man-in-the-middle (MITM) attacks.
- Obtain SSL/TLS certificates from trusted providers like Let’s Encrypt.
- Redirect all HTTP traffic to HTTPS.
const express = require('express');
const https = require('https');
const fs = require('fs');
const app = express();
const options = {
key: fs.readFileSync('server-key.pem'),
cert: fs.readFileSync('server-cert.pem')
};
https.createServer(options, app).listen(443, () => {
console.log('Secure server running on port 443');
});
5. Use Security Headers
HTTP security headers can mitigate certain attacks like clickjacking, cross-site scripting (XSS), and MIME-type sniffing.
- Use the helmet middleware to set security headers:
const helmet = require('helmet');
app.use(helmet());
Recommended headers:
Content-Security-Policy
: Prevent unauthorized script execution.X-Content-Type-Options
: Prevent MIME-type sniffing.Strict-Transport-Security
: Enforce HTTPS connections.
6. Prevent Cross-Site Scripting (XSS)
Escape User Input
Ensure user-provided data is properly escaped before rendering it. Use libraries like DOMPurify or escape HTML entities manually.
Content-Security-Policy
Deploy a strong Content-Security-Policy
header to restrict the execution of scripts and loading of external resources.
7. Rate Limiting and Throttling
Rate limiting helps protect your API from brute-force attacks and abuse.
- Use libraries like express-rate-limit to limit the number of requests a client can make in a certain time period:
const rateLimit = require('express-rate-limit');
const limiter = rateLimit({
windowMs: 15 * 60 * 1000, // 15 minutes
max: 100 // Limit each IP to 100 requests per window
});
app.use('/api/', limiter);
8. Prevent Cross-Site Request Forgery (CSRF)
CSRF attacks trick authenticated users into executing unwanted actions. To prevent this:
- Use csurf middleware to generate and verify CSRF tokens:
const csrf = require('csurf');
app.use(csrf({ cookie: true }));
9. Keep Dependencies Updated
Outdated packages can introduce vulnerabilities into your application. Regularly update dependencies and monitor their security status using tools like:
- npm audit
- Snyk
- Dependabot
10. Use Logging and Monitoring
Monitor your API’s activity to detect and respond to unusual behavior promptly:
- Use logging libraries like winston or bunyan for structured logs.
- Implement real-time monitoring tools like New Relic, Prometheus, or Datadog.
Conclusion
Securing Node.js APIs is a continuous process that requires implementing best practices and keeping up with new security vulnerabilities.
You may also like:
1) How do you optimize a website’s performance?
2) Change Your Programming Habits Before 2025: My Journey with 10 CHALLENGES
3) Senior-Level JavaScript Promise Interview Question
4) What is Database Indexing, and Why is It Important?
5) Can AI Transform the Trading Landscape?
Read more blogs from Here
Share your experiences in the comments, and let’s discuss how to tackle them!
Follow me on Linkedin
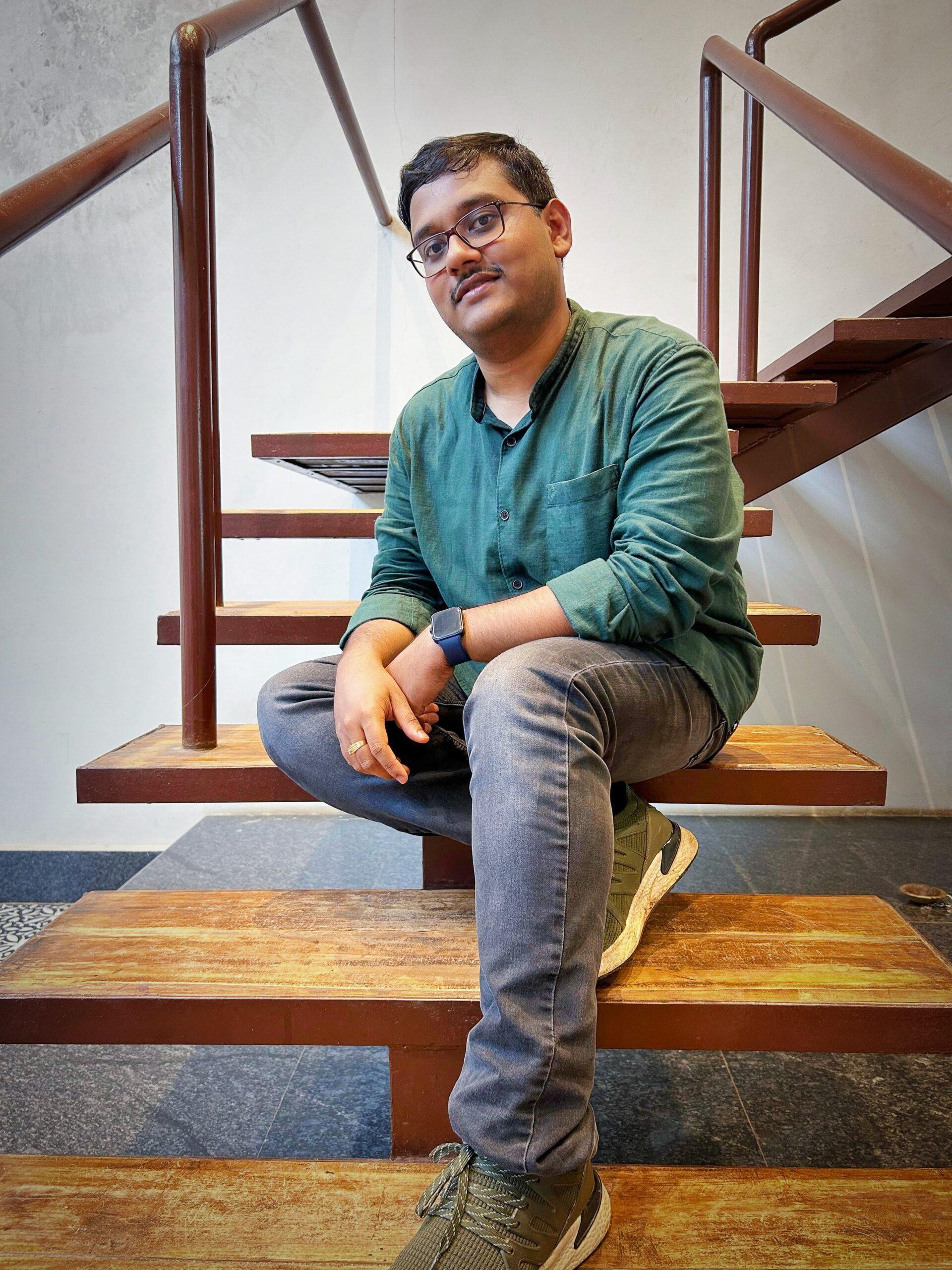
Trust me, I’m a software developer—debugging by day, chilling by night.