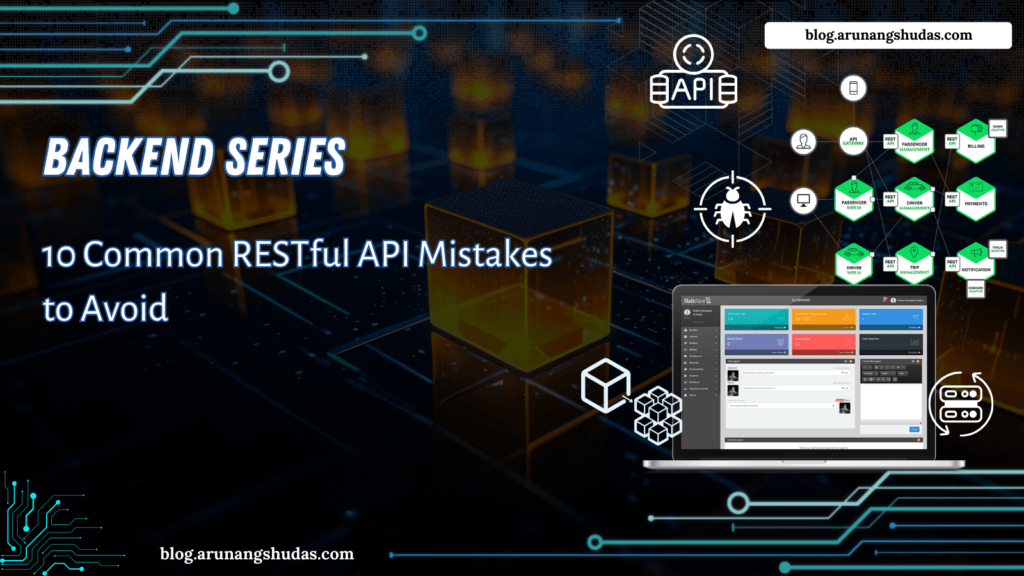
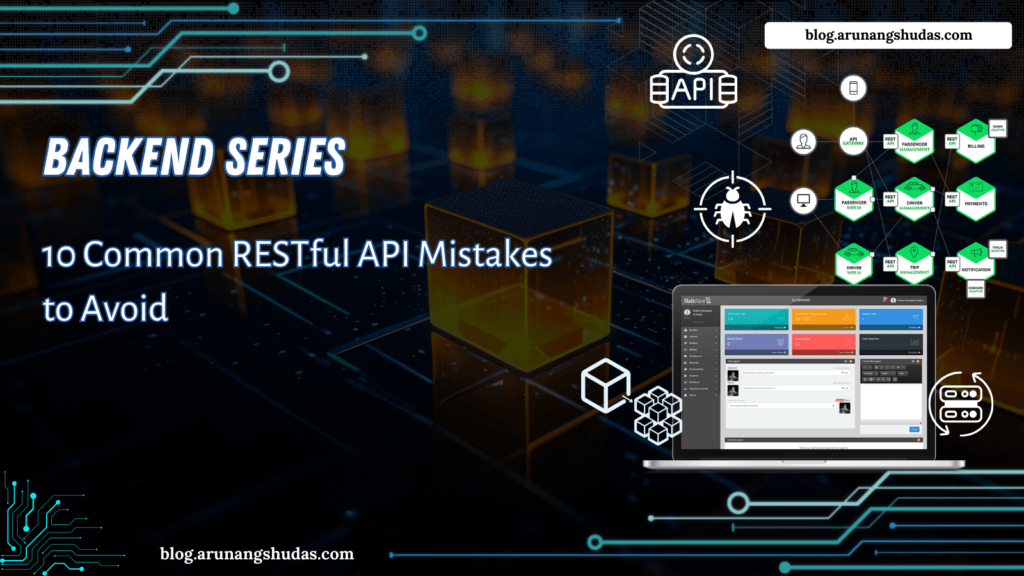
Building RESTful APIs is an essential skill for modern software development. Whether you’re developing a microservice architecture, integrating with third-party applications, or building APIs for mobile apps, REST APIs serve as the backbone of communication between systems.
But here’s the truth: many developers unintentionally make mistakes that lead to poor API performance, security vulnerabilities, or bad developer experience.
So, how can you ensure your RESTful API is well-designed, scalable, and easy to use?
One of the biggest mistakes developers make is misusing HTTP methods. RESTful APIs rely on standard HTTP verbs to perform actions on resources, but developers often mix them up.
Using POST
for fetching data:
POST /users/getAll
This is wrong because POST
is meant for creating resources, not fetching data. Similarly, using GET
for updating data is also incorrect.
Use the correct HTTP methods for specific actions:
HTTP Method | Purpose |
---|---|
GET | Retrieve data |
POST | Create new resources |
PUT | Update/replace resources |
PATCH | Partially update resources |
DELETE | Remove resources |
GET /users # Fetch all users
POST /users # Create a new user
PUT /users/1 # Replace user with ID 1
PATCH /users/1 # Update user with ID 1
DELETE /users/1 # Remove user with ID 1
By following this convention, you make your API intuitive and predictable.
Many developers always return 200 OK
, regardless of the actual outcome. This makes error handling confusing for API consumers.
Returning 200 OK
even when something goes wrong:
{
"status": "error",
"message": "User not found"
}
Use appropriate HTTP status codes:
Status Code | Meaning |
---|---|
200 OK | Success |
201 Created | Resource successfully created |
204 No Content | Successful request, but no response body |
400 Bad Request | Client error (e.g., invalid input) |
401 Unauthorized | Authentication required |
403 Forbidden | Access denied |
404 Not Found | Resource not found |
500 Internal Server Error | Unexpected server failure |
GET /users/999
Response:
{
"error": "User not found"
}
With a proper status code:
HTTP/1.1 404 Not Found
This helps clients handle errors correctly.
Your API might be leaking sensitive data unknowingly, which could lead to security vulnerabilities.
Returning sensitive information in API responses:
{
"id": 1,
"name": "John Doe",
"email": "john@example.com",
"password": "hashed_password",
"token": "abc123"
}
Never expose passwords, authentication tokens, or internal system details. Instead, return only the necessary data.
{
"id": 1,
"name": "John Doe",
"email": "john@example.com"
}
If you need authentication tokens, use secure HTTP headers instead of exposing them in JSON responses.
Fetching too much data in a single request can slow down your API and overload your database.
Returning all users without pagination:
GET /users
Response:
[
{"id": 1, "name": "Alice"},
{"id": 2, "name": "Bob"},
...
{"id": 10000, "name": "Zara"}
]
This is inefficient and can crash your server.
Use pagination to limit the results:
GET /users?page=1&limit=50
Response:
{
"data": [
{"id": 1, "name": "Alice"},
{"id": 2, "name": "Bob"}
],
"total": 10000,
"page": 1,
"limit": 50
}
This makes your API more efficient and scalable.
If you change your API without versioning, you might break existing clients.
Modifying an API endpoint without versioning:
GET /users
Suddenly, clients using the older API might stop working.
Always include a version number in your API:
GET /v1/users
Or use headers for versioning:
GET /users
Accept: application/vnd.myapi.v2+json
This ensures backward compatibility.
Using long, unnecessary nested URLs:
GET /api/v1/users/list/all
Keep URLs simple and readable:
GET /users
RESTful APIs should focus on resources, not actions.
Common security mistakes include:
Generic error messages frustrate users.
Returning this when something goes wrong:
{
"error": "Something went wrong"
}
Give meaningful error messages:
{
"error": "Invalid email format",
"code": "INVALID_EMAIL"
}
If clients need to call multiple endpoints to get basic data, your API is too chatty.
Use query parameters or filtering to return only necessary data.
GET /users?fields=id,name,email
Poor documentation makes API adoption difficult.
Use Swagger/OpenAPI to document your API.
Avoiding these common REST API mistakes will make your APIs faster, more secure, and easier to use. Follow best practices, and your API will be a joy for developers to work with!
You may also like:
1) 5 Common Mistakes in Backend Optimization
2) 7 Tips for Boosting Your API Performance
3) How to Identify Bottlenecks in Your Backend
4) 8 Tools for Developing Scalable Backend Solutions
5) 5 Key Components of a Scalable Backend System
6) 6 Common Mistakes in Backend Architecture Design
7) 7 Essential Tips for Scalable Backend Architecture
8) Token-Based Authentication: Choosing Between JWT and Paseto for Modern Applications
9) API Rate Limiting and Abuse Prevention Strategies in Node.js for High-Traffic APIs
10) Can You Answer This Senior-Level JavaScript Promise Interview Question?
11) 5 Reasons JWT May Not Be the Best Choice
12) 7 Productivity Hacks I Stole From a Principal Software Engineer
13) 7 Common Mistakes in package.json Configuration
Read more blogs from Here
Share your experiences in the comments, and let’s discuss how to tackle them!
Follow me on Linkedin